First you need to register your application in Azure Portal.
Here’s a detailed guide how to do that:
https://docs.microsoft.com/en-us/azure/active-directory/develop/quickstart-register-app
Enable additional flows:
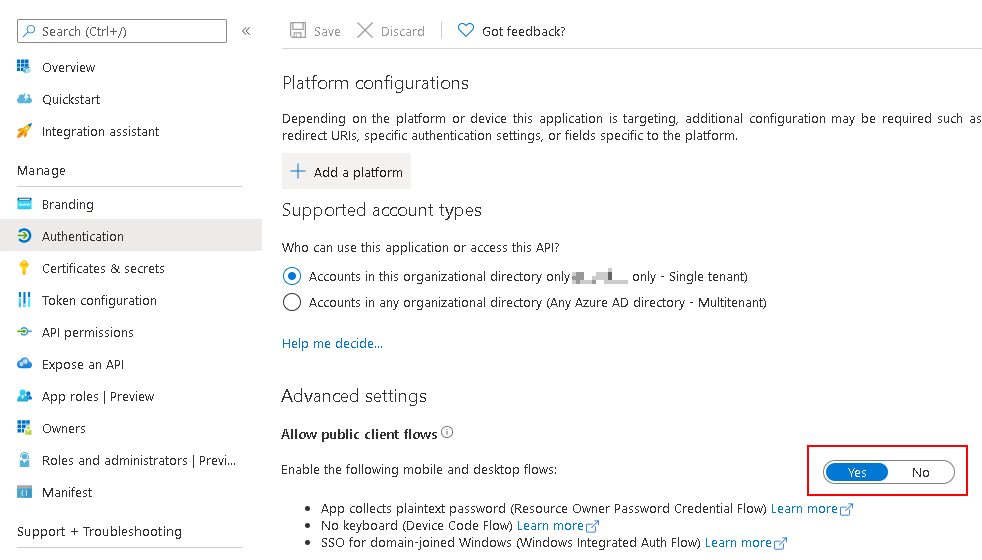
Then you need to apply correct API permissions and grant the admin consent for your domain.

In the API permissions / Add a permission wizard, select Microsoft Graph and then Delegated permissions to find the following permission scopes listed:
- offline_access
- IMAP.AccessAsUser.All
- POP.AccessAsUser.All
- SMTP.Send
Remember to grant admin consent.
Use Microsoft Authentication Library for .NET (MSAL.NET) nuget package to obtain an access token:
https://www.nuget.org/packages/Microsoft.Identity.Client/
string clientId = "Application (client) ID"; string tenantId = "Directory (tenant) ID"; string clientSecret = "Client Secret"; string userEmail = "Username for mailbox"; string userPassword = "Password for that user"; IPublicClientApplication app = PublicClientApplicationBuilder.Create(clientId) .WithAuthority(AzureCloudInstance.AzurePublic, tenantId) .Build(); var scopes = new string[] { "offline_access", "email", "https://outlook.office.com/IMAP.AccessAsUser.All", "https://outlook.office.com/POP.AccessAsUser.All", "https://outlook.office.com/SMTP.Send", };
Now acquire the access token and user email address:
var accounts = await app.GetAccountsAsync(); AuthenticationResult result; if (accounts.Any()) { IAccount account = accounts.FirstOrDefault(); result = await app.AcquireTokenSilent(scopes, account).ExecuteAsync(); } else { SecureString securePassword = new SecureString(); foreach (char c in userPassword) { securePassword.AppendChar(c); } result = await app.AcquireTokenByUsernamePassword( scopes, userEmail, securePassword).ExecuteAsync(); } string user = result.Account.Username; string accessToken = result.AccessToken;
Finally you can connect to IMAP/POP3/SMTP server and authenticate:
using (Imap client = new Imap()) { client.ConnectSSL("outlook.office365.com"); client.LoginOAUTH2(user, accessToken); client.SelectInbox(); // ... client.Close(); }